JavaScript: How to Check if a Key Exists in an Object
JavaScript: How to Check if a Key Exists in an Object
In JavaScript, objects play a crucial role, often used to store collections of data. When working with objects, you might frequently need to determine whether a specific key exists within an object. Checking for the existence of a key is a common task, and there are several ways to achieve this in JavaScript. In this blog post, we’ll explore various methods to check if a key exists in an object, explaining each technique with examples. By the end of this post, you’ll have a solid understanding of how to handle this common requirement efficiently.
Using the in Operator
The in operator is a straightforward and widely-used method to check if a key exists in an object. It returns true if the key is present, either directly on the object or in its prototype chain.
let person = {
name: ‘John’,
age: 30
};
console.log(‘name’ in person); // true
console.log(‘address’ in person); // false
In the example above, the in operator checks for the existence of the keys ‘name’ and ‘address’ in the person object. It correctly returns true for ‘name’ and false for ‘address’.
Using the hasOwnProperty Method
The hasOwnProperty method is another reliable way to check if a key exists in an object. Unlike the in operator, hasOwnProperty does not check the prototype chain, only the object itself.
let person = {
name: ‘John’,
age: 30
};
console.log(person.hasOwnProperty(‘name’)); // true
console.log(person.hasOwnProperty(‘address’)); // false
Here, the hasOwnProperty method checks only the person object itself for the keys ‘name’ and ‘address’, returning true and false, respectively.
Using the undefined Comparison
Another simple way to check for the existence of a key is to compare it to undefined. This method involves accessing the key directly and checking if it is undefined.
let person = {
name: ‘John’,
age: 30
};
console.log(http://person.name !== undefined); // true
console.log(person.address !== undefined); // false
While this method is straightforward, it can be less reliable if the object’s keys can have undefined values. Therefore, use this technique with caution and consider your specific use case.
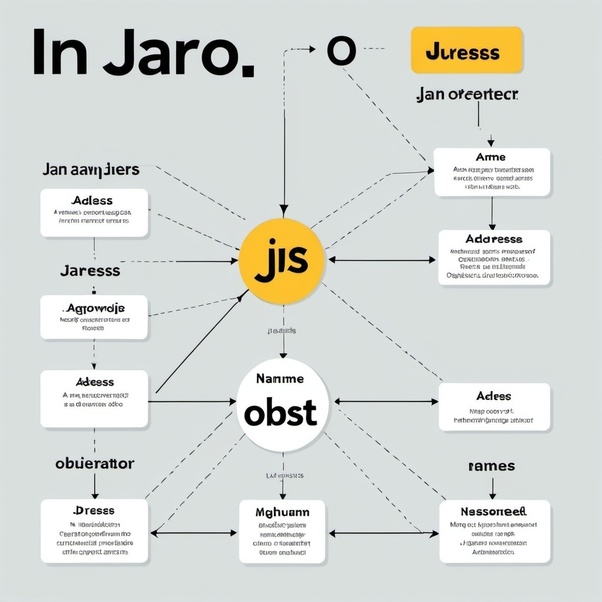
Using the Object.keys Method
The Object.keys method returns an array of a given object’s own enumerable property names. You can use it to check for the existence of a key by checking if the key is present in the array.
let person = {
name: ‘John’,
age: 30
};
console.log(Object.keys(person).includes(‘name’)); // true
console.log(Object.keys(person).includes(‘address’)); // false
In this example, Object.keys(person) returns [‘name’, ‘age’], and the includes method checks for the presence of ‘name’ and ‘address’, returning true and false, respectively.
Using Map Objects for Key Existence Checks
In modern JavaScript development, Map objects are often used to store key-value pairs. Map objects provide a has method, which can be used to check if a key exists.
let personMap = new Map();
personMap.set(‘name’, ‘John’);
personMap.set(‘age’, 30);
console.log(personMap.has(‘name’)); // true
console.log(personMap.has(‘address’)); // false
The has method of the Map object provides a clean and efficient way to check for the existence of keys.
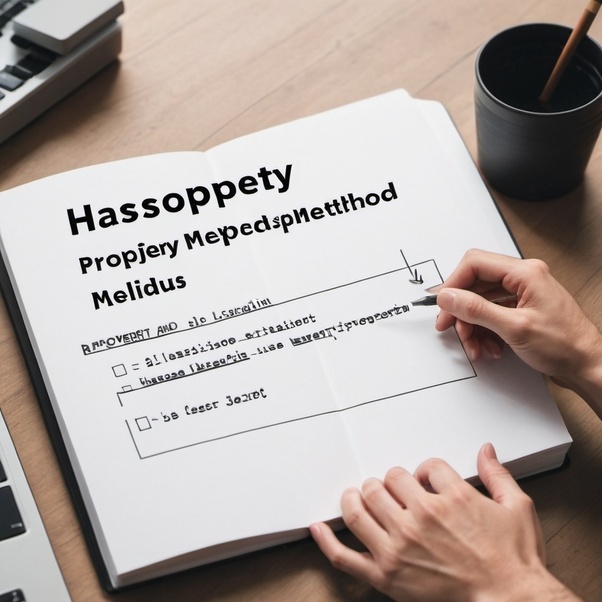
Using Optional Chaining (ES2020)
With the introduction of optional chaining in ES2020, you can safely check for the existence of nested keys without worrying about causing errors if an intermediate key is null or undefined.
let person = {
name: ‘John’,
age: 30,
address: {
city: ‘New York’
}
};
console.log(person?.address?.city !== undefined); // true
console.log(person?.address?.zipCode !== undefined); // false
console.log(person?.contact?.phone !== undefined); // false
Optional chaining provides a concise and readable way to check for the existence of nested keys while avoiding errors.
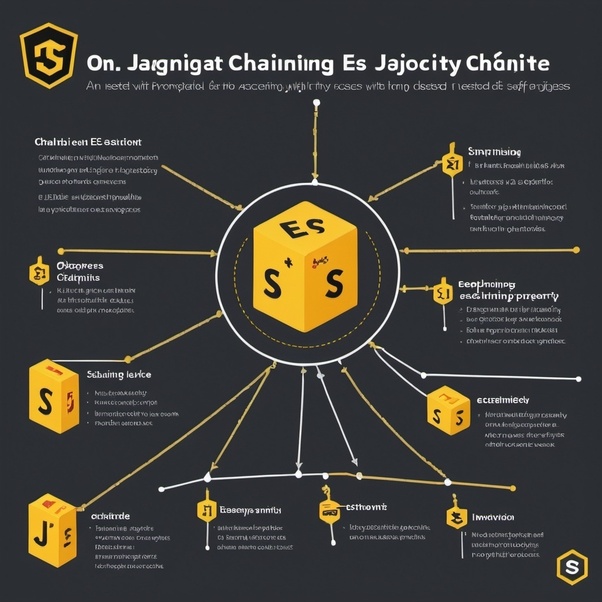
Conclusion
In JavaScript, there are multiple ways to check if a key exists in an object. Each method has its own advantages and use cases:
in Operator: Checks for keys in the object and its prototype chain.
hasOwnProperty Method: Checks only the object’s own properties.
undefined Comparison: Simple but less reliable if keys can have undefined values.
Object.keys Method: Converts keys to an array and checks for their presence.
Map Objects: Uses the has method for clean key existence checks.
Optional Chaining: Safely checks for nested keys introduced in ES2020.
By understanding and using these methods, you can handle key existence checks in JavaScript effectively, ensuring your code is robust and reliable.
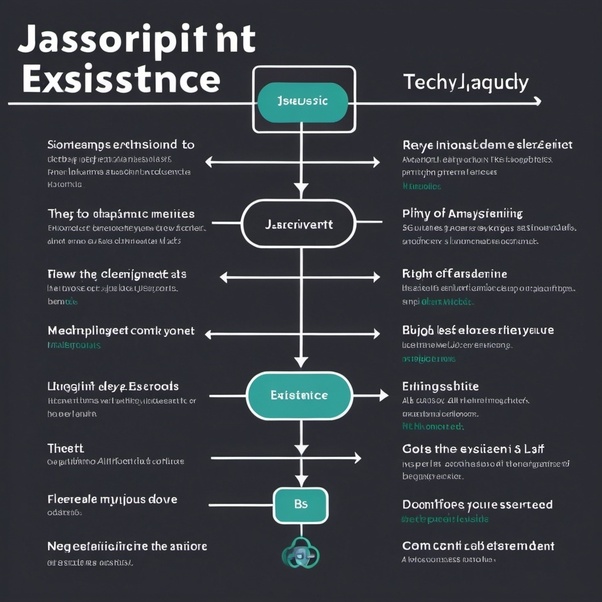
If you’re looking for professional JavaScript development services, Front Page – TechInn Global can help. Our team of experienced developers specializes in building robust, efficient, and scalable JavaScript solutions tailored to your business needs. Whether you need help with front-end development, back-end systems, or custom JavaScript functionalities, we have the expertise to deliver high-quality results. Contact us today to learn more about how our services can help you achieve your development goals. Visit Front Page – TechInn Global for more information and to see how we can assist you with your JavaScript projects.
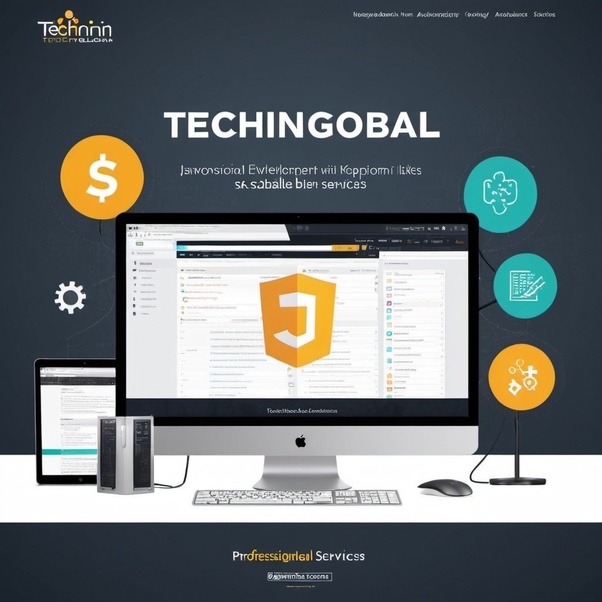